Preparing for a security testing interview can be daunting, but having a solid grasp of key questions and answers can make all the difference. This article compiles 25 essential security testing interview questions and answers to help you ace your next interview with confidence. Dive in to enhance your understanding and readiness for the big day.
What are Security Testing interview questions?
Security Testing interview questions are designed to evaluate a candidate's knowledge and skills in identifying vulnerabilities, threats, and risks within software systems. These questions often cover topics such as penetration testing, security protocols, and incident response strategies to ensure the candidate can effectively safeguard an organization's digital assets.
Why do interviewers ask Security Testing questions?
The main purpose of Security Testing interview questions is to assess a candidate's ability to identify and mitigate potential vulnerabilities within software systems. Interviewers ask these questions to ensure that the candidate possesses the necessary skills to protect an organization's digital assets from threats and breaches.
25 Security Testing interview questions
Here are some essential security testing interview questions to help you prepare:
- What is security testing, and why is it important in software development?
- Can you explain the difference between static and dynamic security testing?
- Describe the OWASP Top Ten and its significance in security testing.
- What are some common security vulnerabilities found in web applications?
- How would you perform a penetration test on a web application?
- Write a simple script to check for SQL injection vulnerabilities in a given input.
- Explain the concept of Cross-Site Scripting (XSS) and how to prevent it.
- What tools do you use for security testing, and why?
- Describe the process of threat modeling and its importance in security testing.
- Write a function in Python that checks if a given password meets security criteria (length, complexity, etc.).
- What is the role of encryption in securing data, and how would you implement it?
- Explain the concept of session management and its security implications.
- Write a code snippet to demonstrate how to securely store passwords using hashing.
- What is a security policy, and what key elements should it include?
- Describe how you would test for insecure direct object references (IDOR).
- Write a JavaScript function that sanitizes user input to prevent XSS attacks.
- What is the difference between authentication and authorization?
- How would you approach testing an API for security vulnerabilities?
- Write a simple program that simulates a brute-force attack on a login system.
- Explain the concept of a security audit and its components.
- What are some best practices for secure coding?
- Write a code snippet to demonstrate how to implement rate limiting to prevent DoS attacks.
- How do you stay updated on the latest security threats and vulnerabilities?
- Describe the importance of logging and monitoring in security testing.
- Write a function that checks for common security misconfigurations in a web server setup.
1. What is security testing, and why is it important in software development?
Why you might get asked this: Interviewers often ask "What is security testing, and why is it important in software development?" to gauge your foundational understanding of security principles and their critical role in protecting software systems, which is essential for roles such as Security Analyst or Software Developer.
How to answer:
- Define security testing as the process of identifying and mitigating vulnerabilities in software systems.
- Emphasize its importance in protecting sensitive data and ensuring system integrity.
- Highlight how it helps in maintaining user trust and compliance with regulatory standards.
Example answer:
"Security testing is the process of identifying and mitigating vulnerabilities in software systems to protect sensitive data and ensure system integrity. It is crucial in software development as it helps maintain user trust and compliance with regulatory standards."
2. Can you explain the difference between static and dynamic security testing?
Why you might get asked this: Interviewers ask "Can you explain the difference between static and dynamic security testing?" to assess your understanding of different testing methodologies and their applications, which is crucial for roles such as Security Engineer or QA Tester.
How to answer:
- Define static security testing as the analysis of code without executing it.
- Explain dynamic security testing as the evaluation of a running application to identify vulnerabilities.
- Highlight the complementary nature of both methods in providing a comprehensive security assessment.
Example answer:
"Static security testing involves analyzing the source code without executing it, allowing for early detection of vulnerabilities. Dynamic security testing, on the other hand, evaluates the application during runtime to identify potential security issues in a live environment."
3. Describe the OWASP Top Ten and its significance in security testing.
Why you might get asked this: Interviewers ask "Describe the OWASP Top Ten and its significance in security testing" to evaluate your knowledge of common security vulnerabilities and best practices, which is essential for roles such as Security Analyst or Penetration Tester.
How to answer:
- Briefly explain that the OWASP Top Ten is a list of the most critical web application security risks.
- Highlight its role in guiding developers and security professionals in identifying and mitigating these risks.
- Emphasize its importance in establishing industry standards and best practices for web security.
Example answer:
"The OWASP Top Ten is a list of the most critical web application security risks, serving as a valuable resource for developers and security professionals. Its significance lies in guiding the industry towards best practices and standards for web security."
4. What are some common security vulnerabilities found in web applications?
Why you might get asked this: Interviewers ask "What are some common security vulnerabilities found in web applications?" to assess your familiarity with prevalent security issues and your ability to identify and address them, which is crucial for roles such as Web Developer or Security Engineer.
How to answer:
- Mention common vulnerabilities such as SQL injection, Cross-Site Scripting (XSS), and Cross-Site Request Forgery (CSRF).
- Briefly explain the impact of each vulnerability on web applications.
- Highlight the importance of regular security testing and code reviews to mitigate these risks.
Example answer:
"Common security vulnerabilities in web applications include SQL injection, Cross-Site Scripting (XSS), and Cross-Site Request Forgery (CSRF). These vulnerabilities can lead to unauthorized data access, data manipulation, and compromised user sessions, making regular security testing and code reviews essential."
5. How would you perform a penetration test on a web application?
Why you might get asked this: Interviewers ask "How would you perform a penetration test on a web application?" to evaluate your practical skills in identifying and exploiting vulnerabilities, which is crucial for roles such as Penetration Tester or Security Consultant.
How to answer:
- Outline the initial steps of reconnaissance and information gathering.
- Describe the process of identifying and exploiting vulnerabilities.
- Emphasize the importance of documenting findings and providing remediation recommendations.
Example answer:
"To perform a penetration test on a web application, I would start with reconnaissance and information gathering to understand the application's structure and potential entry points. Then, I would identify and exploit vulnerabilities, documenting my findings and providing remediation recommendations."
6. Write a simple script to check for SQL injection vulnerabilities in a given input.
Why you might get asked this: Interviewers ask "Write a simple script to check for SQL injection vulnerabilities in a given input" to assess your practical coding skills and understanding of security threats, which is crucial for roles such as Security Engineer or Software Developer.
How to answer:
- Explain the purpose of the script in identifying SQL injection vulnerabilities.
- Describe the basic structure of the script, including input validation and query execution.
- Highlight the importance of testing the script against various inputs to ensure its effectiveness.
Example answer:
"To check for SQL injection vulnerabilities, you can write a script that tests user inputs against common SQL injection patterns. Here's a simple example in Python:"
def check_sql_injection(input_string):
sql_injection_patterns = ["'", "--", ";", "/*", "*/", "@@", "@", "char", "nchar", "varchar", "nvarchar", "alter", "begin", "cast", "create", "cursor", "declare", "delete", "drop", "end", "exec", "execute", "fetch", "insert", "kill", "select", "sys", "sysobjects", "syscolumns", "table", "update"]
for pattern in sql_injection_patterns:
if pattern.lower() in input_string.lower():
return True
return False
7. Explain the concept of Cross-Site Scripting (XSS) and how to prevent it.
Why you might get asked this: Interviewers ask "Explain the concept of Cross-Site Scripting (XSS) and how to prevent it" to evaluate your understanding of common web application vulnerabilities and your ability to implement security measures, which is crucial for roles such as Web Developer or Security Engineer.
How to answer:
- Define Cross-Site Scripting (XSS) as a vulnerability that allows attackers to inject malicious scripts into web pages viewed by users.
- Explain that XSS can lead to data theft, session hijacking, and other malicious activities.
- Highlight prevention techniques such as input validation, output encoding, and using security headers like Content Security Policy (CSP).
Example answer:
"Cross-Site Scripting (XSS) is a vulnerability that allows attackers to inject malicious scripts into web pages viewed by users, potentially leading to data theft and session hijacking. To prevent XSS, implement input validation, output encoding, and use security headers like Content Security Policy (CSP)."
8. What tools do you use for security testing, and why?
Why you might get asked this: Interviewers ask "What tools do you use for security testing, and why?" to assess your familiarity with industry-standard tools and your ability to effectively utilize them in identifying and mitigating security vulnerabilities, which is crucial for roles such as Security Engineer or Penetration Tester.
How to answer:
- Mention specific tools like Burp Suite, OWASP ZAP, and Nessus.
- Briefly explain the primary function of each tool.
- Highlight why you prefer these tools based on their features and effectiveness.
Example answer:
"I use tools like Burp Suite, OWASP ZAP, and Nessus for security testing. These tools are effective in identifying vulnerabilities, providing comprehensive reports, and offering features that streamline the testing process."
9. Describe the process of threat modeling and its importance in security testing.
Why you might get asked this: Interviewers ask "Describe the process of threat modeling and its importance in security testing" to evaluate your ability to systematically identify and address potential security threats, which is crucial for roles such as Security Analyst or Software Developer, for example.
How to answer:
- Define threat modeling as a structured approach to identifying and mitigating potential security threats.
- Explain the steps involved, such as identifying assets, potential threats, and vulnerabilities.
- Highlight its importance in proactively addressing security risks and improving overall system security.
Example answer:
"Threat modeling is a structured approach to identifying and mitigating potential security threats by analyzing assets, threats, and vulnerabilities. It is crucial in proactively addressing security risks and enhancing overall system security."
10. Write a function in Python that checks if a given password meets security criteria (length, complexity, etc.).
Why you might get asked this: Interviewers ask "Write a function in Python that checks if a given password meets security criteria (length, complexity, etc.)" to evaluate your practical coding skills and understanding of security best practices, which is crucial for roles such as Security Engineer or Software Developer, for example.
How to answer:
- Explain the importance of password security criteria such as length and complexity.
- Describe the function's logic, including checks for length, uppercase, lowercase, digits, and special characters.
- Highlight the use of regular expressions or built-in string methods to implement these checks efficiently.
Example answer:
"An amazing answer would include a Python function that checks for password length, uppercase, lowercase, digits, and special characters. It should efficiently use regular expressions to validate these criteria."
import re
def is_secure_password(password):
if len(password) < 8:
return False
if not re.search("[A-Z]", password):
return False
if not re.search("[a-z]", password):
return False
if not re.search("[0-9]", password):
return False
if not re.search("[@#$%^&+=]", password):
return False
return True
11. What is the role of encryption in securing data, and how would you implement it?
Why you might get asked this: Interviewers ask "What is the role of encryption in securing data, and how would you implement it?" to assess your understanding of data protection mechanisms and practical implementation skills, which is crucial for roles such as Security Engineer or Software Developer, for example.
How to answer:
- Define encryption as the process of converting data into a coded format to prevent unauthorized access.
- Explain its role in protecting sensitive information during storage and transmission.
- Describe the implementation using algorithms like AES or RSA and the importance of key management.
Example answer:
"Encryption is the process of converting data into a coded format to prevent unauthorized access, ensuring data confidentiality and integrity. To implement it, I would use algorithms like AES or RSA and emphasize the importance of proper key management."
12. Explain the concept of session management and its security implications.
Why you might get asked this: Interviewers ask "Explain the concept of session management and its security implications" to assess your understanding of maintaining secure user sessions and preventing attacks like session hijacking, which is crucial for roles such as Security Engineer or Web Developer, for example.
How to answer:
- Define session management as the process of securely handling user sessions in web applications.
- Explain its importance in maintaining user authentication and authorization throughout a session.
- Highlight common security measures like using secure cookies, session timeouts, and regenerating session IDs.
Example answer:
"Session management is the process of securely handling user sessions in web applications, ensuring that user authentication and authorization are maintained throughout a session. It involves using secure cookies, session timeouts, and regenerating session IDs to prevent attacks like session hijacking."
13. Write a code snippet to demonstrate how to securely store passwords using hashing.
Why you might get asked this: Interviewers ask "Write a code snippet to demonstrate how to securely store passwords using hashing" to evaluate your practical coding skills and understanding of secure password storage practices, which is crucial for roles such as Security Engineer or Software Developer, for example.
How to answer:
- Explain the importance of hashing passwords to protect against unauthorized access.
- Describe the use of a strong hashing algorithm like bcrypt or Argon2.
- Highlight the necessity of adding a unique salt to each password before hashing.
Example answer:
"An amazing answer would include a Python code snippet that demonstrates the use of bcrypt for hashing passwords. It should also highlight the importance of salting the passwords to enhance security."
import bcrypt
def hash_password(password):
salt = bcrypt.gensalt()
hashed = bcrypt.hashpw(password.encode('utf-8'), salt)
return hashed
14. What is a security policy, and what key elements should it include?
Why you might get asked this: Interviewers ask "What is a security policy, and what key elements should it include?" to evaluate your understanding of organizational security frameworks and your ability to develop comprehensive security guidelines, which is crucial for roles such as Security Manager or IT Administrator, for example.
How to answer:
- Define a security policy as a set of rules and practices for protecting an organization's digital assets.
- Highlight key elements such as access control, data protection, and incident response.
- Emphasize the importance of regular updates and employee training to ensure policy effectiveness.
Example answer:
"A security policy is a set of rules and practices designed to protect an organization's digital assets. Key elements include access control, data protection, and incident response, with regular updates and employee training to ensure effectiveness."
15. Describe how you would test for insecure direct object references (IDOR).
Why you might get asked this: Interviewers ask "Describe how you would test for insecure direct object references (IDOR)" to evaluate your ability to identify and mitigate access control vulnerabilities, which is crucial for roles such as Security Engineer or Penetration Tester, for example.
How to answer:
- Explain that IDOR testing involves verifying if users can access objects without proper authorization.
- Describe using automated tools and manual testing to identify potential IDOR vulnerabilities.
- Highlight the importance of reviewing access control mechanisms and ensuring proper authorization checks.
Example answer:
"An amazing answer would involve verifying if users can access objects without proper authorization by using automated tools and manual testing. It should also highlight the importance of reviewing access control mechanisms and ensuring proper authorization checks."
16. Write a JavaScript function that sanitizes user input to prevent XSS attacks.
Why you might get asked this: Interviewers ask "Write a JavaScript function that sanitizes user input to prevent XSS attacks" to evaluate your practical coding skills and understanding of web security practices, which is crucial for roles such as Web Developer or Security Engineer, for example.
How to answer:
- Explain the importance of sanitizing user input to prevent XSS attacks.
- Describe the use of built-in JavaScript functions to escape special characters.
- Highlight the necessity of validating and encoding user input before rendering it on the web page.
Example answer:
"An amazing answer would include a JavaScript function that escapes special characters to prevent XSS attacks. It should also highlight the importance of validating and encoding user input before rendering it on the web page."
function sanitizeInput(input) {
return input.replace(/[&<>"'\/]/g, function (char) {
const escapeChars = {
'&': '&',
'<': '<',
'>': '>',
'"': '"',
"'": ''',
'/': '/'
};
return escapeChars[char];
});
}
17. What is the difference between authentication and authorization?
Why you might get asked this: Interviewers ask "What is the difference between authentication and authorization?" to assess your understanding of fundamental security concepts, which is crucial for roles such as Security Engineer or Software Developer, for example.
How to answer:
- Define authentication as the process of verifying a user's identity.
- Explain authorization as the process of granting access to resources based on the user's identity.
- Highlight that authentication occurs before authorization in the security process.
Example answer:
"An amazing answer would define authentication as the process of verifying a user's identity and authorization as the process of granting access to resources based on the user's identity. It should also highlight that authentication occurs before authorization in the security process."
18. How would you approach testing an API for security vulnerabilities?
Why you might get asked this: Interviewers ask "How would you approach testing an API for security vulnerabilities?" to evaluate your practical skills in identifying and mitigating security risks in APIs, which is crucial for roles such as Security Engineer or API Developer, for example.
How to answer:
- Start by outlining the importance of understanding the API's functionality and endpoints.
- Describe using automated tools and manual testing to identify common vulnerabilities like injection attacks and improper authentication.
- Highlight the necessity of reviewing API documentation and implementing security best practices throughout the testing process.
Example answer:
"An amazing answer would start by outlining the importance of understanding the API's functionality and endpoints. It should also describe using automated tools and manual testing to identify common vulnerabilities like injection attacks and improper authentication."
19. Write a simple program that simulates a brute-force attack on a login system.
Why you might get asked this: Interviewers ask "Write a simple program that simulates a brute-force attack on a login system" to evaluate your understanding of attack methodologies and your ability to implement security measures, which is crucial for roles such as Security Engineer or Penetration Tester, for example.
How to answer:
- Explain the concept of a brute-force attack as systematically trying all possible combinations to guess a password.
- Describe the basic structure of the program, including looping through potential passwords and checking them against the target password.
- Highlight the importance of implementing security measures like account lockout and rate limiting to prevent such attacks.
Example answer:
"An amazing answer would explain the concept of a brute-force attack as systematically trying all possible combinations to guess a password. It should also describe the basic structure of the program, including looping through potential passwords and checking them against the target password."
def brute_force_attack(target_password, password_list):
for password in password_list:
if password == target_password:
return True
return False
20. Explain the concept of a security audit and its components.
Why you might get asked this: Interviewers ask "Explain the concept of a security audit and its components" to assess your understanding of comprehensive security evaluations and your ability to identify and address potential vulnerabilities, which is crucial for roles such as Security Analyst or IT Auditor, for example.
How to answer:
- Define a security audit as a systematic evaluation of an organization's security policies and practices.
- Explain that it involves assessing compliance with security standards and identifying vulnerabilities.
- Highlight the key components such as risk assessment, policy review, and technical testing.
Example answer:
"A security audit is a systematic evaluation of an organization's security policies and practices, ensuring compliance with security standards and identifying vulnerabilities. Key components include risk assessment, policy review, and technical testing."
21. What are some best practices for secure coding?
Why you might get asked this: Interviewers ask "What are some best practices for secure coding?" to evaluate your understanding of essential coding principles that prevent security vulnerabilities, which is crucial for roles such as Software Developer or Security Engineer, for example.
How to answer:
- Emphasize the importance of input validation to prevent injection attacks.
- Highlight the use of secure coding libraries and frameworks.
- Mention the necessity of regular code reviews and security testing.
Example answer:
"An amazing answer would emphasize the importance of input validation to prevent injection attacks and highlight the use of secure coding libraries and frameworks. It should also mention the necessity of regular code reviews and security testing."
22. Write a code snippet to demonstrate how to implement rate limiting to prevent DoS attacks.
Why you might get asked this: Interviewers ask "Write a code snippet to demonstrate how to implement rate limiting to prevent DoS attacks" to assess your practical coding skills and understanding of security measures to mitigate denial-of-service attacks, which is crucial for roles such as Security Engineer or Backend Developer, for example.
How to answer:
- Explain the concept of rate limiting as a technique to control the number of requests a user can make to a server.
- Describe using a token bucket algorithm or a fixed window counter to implement rate limiting.
- Highlight the importance of configuring appropriate thresholds to balance security and user experience.
Example answer:
"An amazing answer would explain the concept of rate limiting as a technique to control the number of requests a user can make to a server. It should also describe using a token bucket algorithm or a fixed window counter to implement rate limiting."
from time import time, sleep
class RateLimiter:
def __init__(self, max_requests, period):
self.max_requests = max_requests
self.period = period
self.requests = []
def allow_request(self):
current_time = time()
self.requests = [req for req in self.requests if req > current_time - self.period]
if len(self.requests) < self.max_requests:
self.requests.append(current_time)
return True
return False
# Example usage
limiter = RateLimiter(5, 60) # 5 requests per 60 seconds
for i in range(10):
if limiter.allow_request():
print("Request allowed")
else:
print("Rate limit exceeded")
sleep(10)
23. How do you stay updated on the latest security threats and vulnerabilities?
Why you might get asked this: Interviewers ask "How do you stay updated on the latest security threats and vulnerabilities?" to assess your commitment to continuous learning and staying informed about evolving security challenges, which is crucial for roles such as Security Analyst or Penetration Tester, for example.
How to answer:
- Mention subscribing to reputable security blogs and newsletters.
- Highlight participating in online forums and communities.
- Emphasize attending industry conferences and webinars.
Example answer:
"An amazing answer would mention subscribing to reputable security blogs and newsletters, and highlight participating in online forums and communities. It should also emphasize attending industry conferences and webinars."
24. Describe the importance of logging and monitoring in security testing.
Why you might get asked this: Interviewers ask "Describe the importance of logging and monitoring in security testing" to assess your understanding of proactive security measures and your ability to detect and respond to security incidents, which is crucial for roles such as Security Analyst or IT Administrator, for example.
How to answer:
- Explain that logging and monitoring are essential for detecting and responding to security incidents in real-time.
- Highlight their role in providing a detailed audit trail for forensic analysis and compliance purposes.
- Emphasize the importance of continuous monitoring to identify and mitigate potential threats promptly.
Example answer:
"An amazing answer would explain that logging and monitoring are essential for detecting and responding to security incidents in real-time. It should also highlight their role in providing a detailed audit trail for forensic analysis and compliance purposes."
25. Write a function that checks for common security misconfigurations in a web server setup.
Why you might get asked this: Interviewers ask "Write a function that checks for common security misconfigurations in a web server setup" to evaluate your practical coding skills and understanding of server security best practices, which is crucial for roles such as Security Engineer or System Administrator, for example.
How to answer:
- Explain the importance of identifying common security misconfigurations in web servers.
- Describe the function's logic, including checks for default credentials, open ports, and outdated software.
- Highlight the use of automated tools and scripts to streamline the detection process.
Example answer:
"An amazing answer would include a function that checks for default credentials, open ports, and outdated software in a web server setup. It should also highlight the importance of using automated tools and scripts to streamline the detection process."
def check_security_misconfigurations(server_config):
misconfigurations = []
if server_config.get('default_credentials'):
misconfigurations.append('Default credentials are being used.')
if server_config.get('open_ports'):
misconfigurations.append('There are open ports that should be closed.')
if server_config.get('outdated_software'):
misconfigurations.append('Outdated software versions detected.')
return misconfigurations
Tips to prepare for Security Testing questions
- Understand Common Vulnerabilities: Familiarize yourself with common security vulnerabilities such as SQL injection, XSS, and CSRF. Knowing how to identify and mitigate these issues is crucial.
- Master Security Tools: Gain hands-on experience with industry-standard security testing tools like Burp Suite, OWASP ZAP, and Nessus. Be prepared to discuss how you use these tools in real-world scenarios.
- Stay Updated: Keep up with the latest security trends and threats by following reputable security blogs, participating in forums, and attending webinars. This shows your commitment to continuous learning.
- Practice Coding: Be ready to write and explain code snippets that demonstrate your ability to implement security measures, such as input validation, encryption, and hashing.
- Know Security Frameworks: Understand and be able to discuss security frameworks and guidelines like OWASP Top Ten and NIST. This knowledge is essential for establishing best practices in security testing.
Ace your interview with Final Round AI
If you need help with any of your other interviews, consider signing up for Final Round AI. With its comprehensive suite of AI-driven tools, including the Interview Copilot, AI Resume Builder, and AI Mock Interview, Final Round AI provides real-time actionable guidance to help you ace your interviews. Join the thousands of successful users who have landed their dream jobs with the help of Final Round AI. Sign up now and take the next step towards your career success.
Table of Contents
Related articles
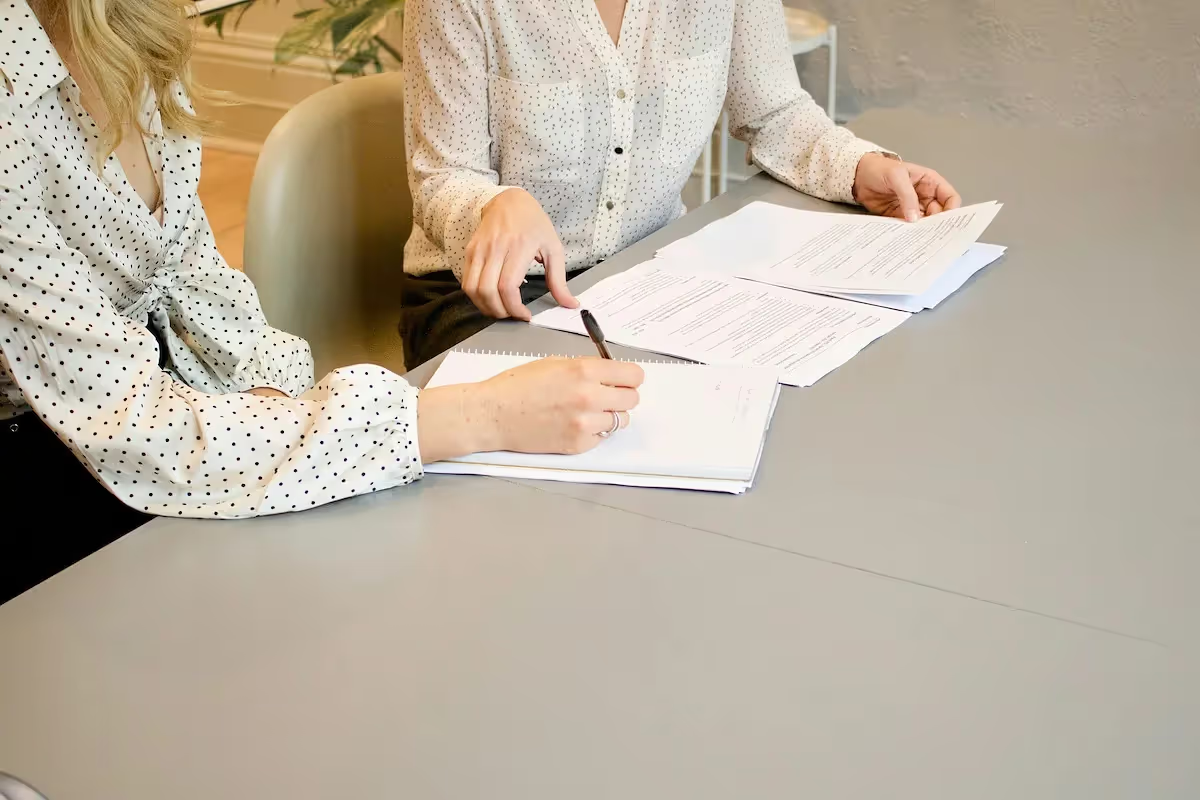
DBMS-Interview Interview Questions (With Answers)
Discover the top 25 DBMS interview questions to ace your next job interview. Prepare effectively with our comprehensive guide.
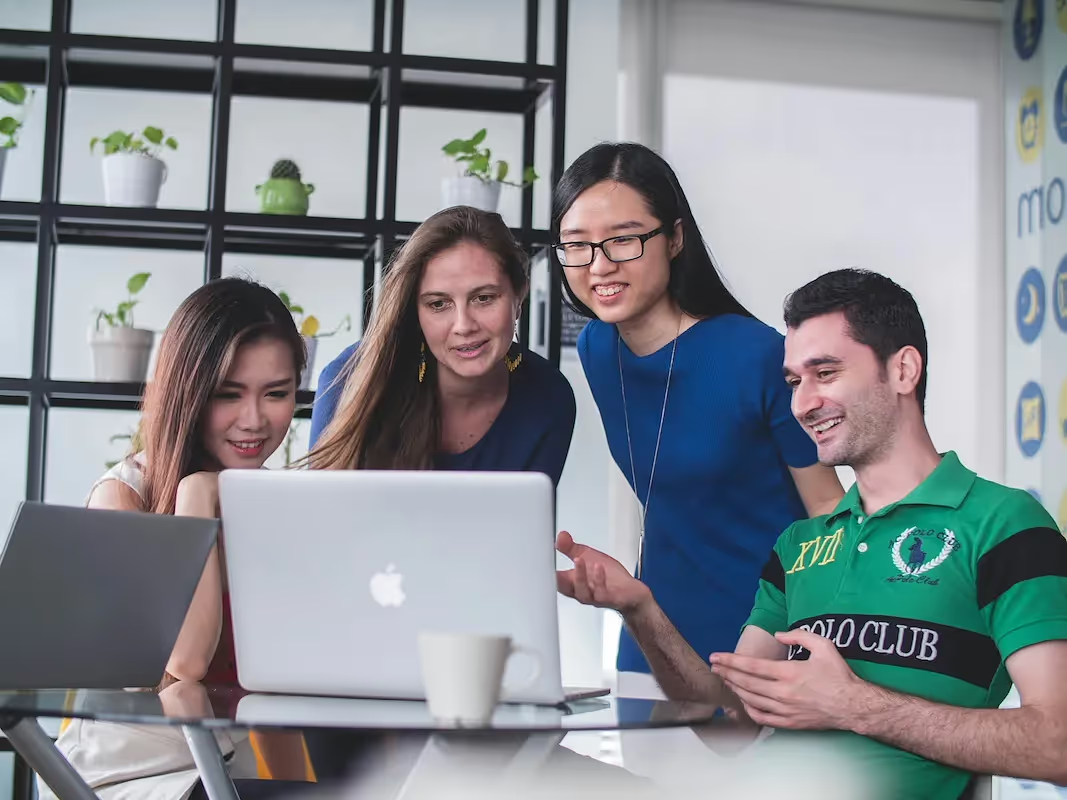
API Design Interview Questions (With Answers)
Discover the top 25 API design interview questions to help you prepare and ace your next tech interview. Essential insights and tips included!

GCP BigQuery Interview Questions (With Answers)
Discover the top 25 GCP BigQuery interview questions to ace your next data engineering job interview. Prepare with confidence!
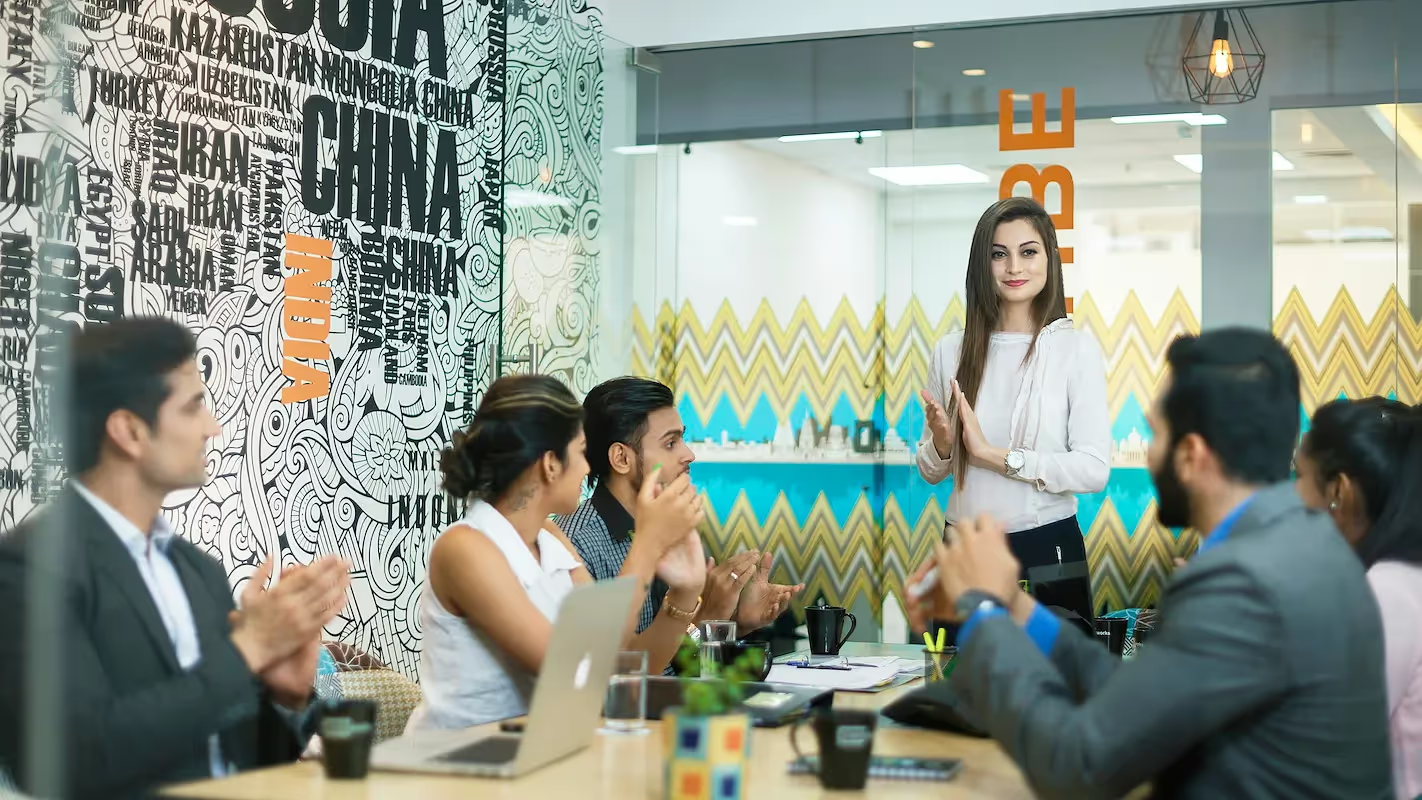
Java Programming Interview Questions (With Answers)
Discover the top 25 Java interview questions to ace your next coding interview. Prepare effectively with our comprehensive guide.